Python ToDo App
This Python project is a command-line task manager that allows users to interactively manage their to-do list. Users can add, view, edit, and complete tasks through a simple menu-driven interface. The program runs in an infinite loop, continuously offering these options until the user chooses to exit. This task manager is designed to be user-friendly and provides a straightforward way for individuals to keep track of their daily tasks directly from the terminal.

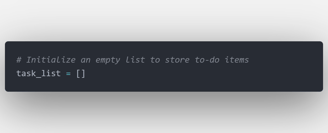
This line initializes an empty list called 'task_list' that will store the tasks or to-do items.
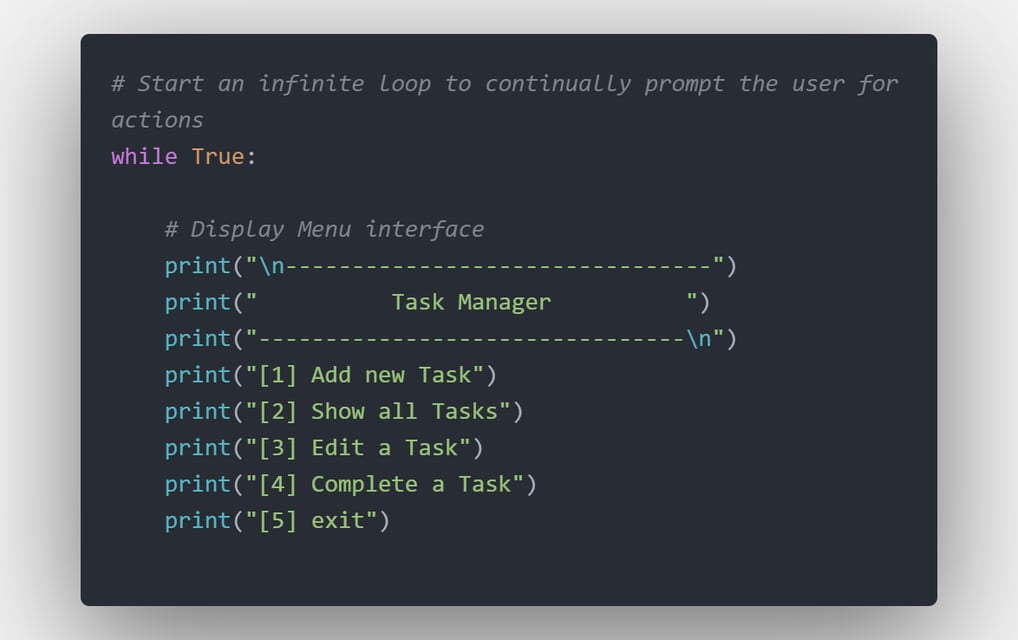
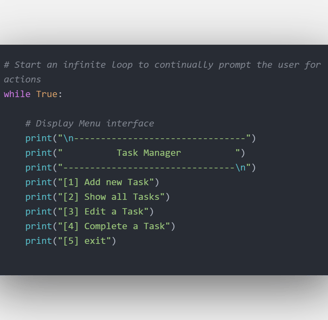
The program enters an infinite loop, which means it will continuously execute the loop's contents until explicitly told to exit (i.e., when the user selects the quit option). This also displays the menu interface to the user, providing options to manage tasks such as adding tasks, showing tasks, editing tasks, completing tasks, and exiting the program.
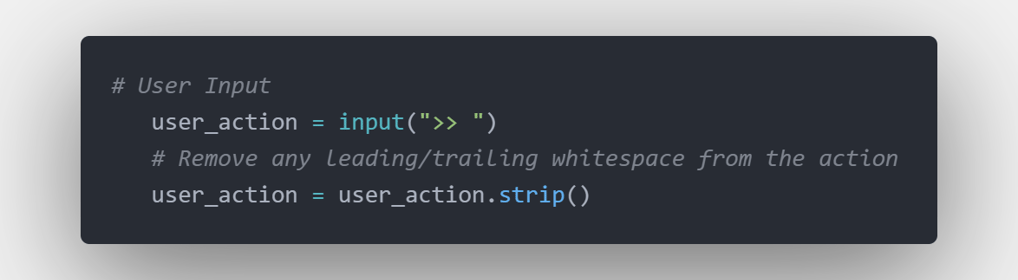
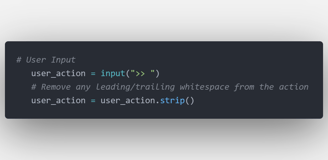
Here, the program prompts the user to enter their choice based on the menu options and removes any extra whitespace from the input for cleaner processing.
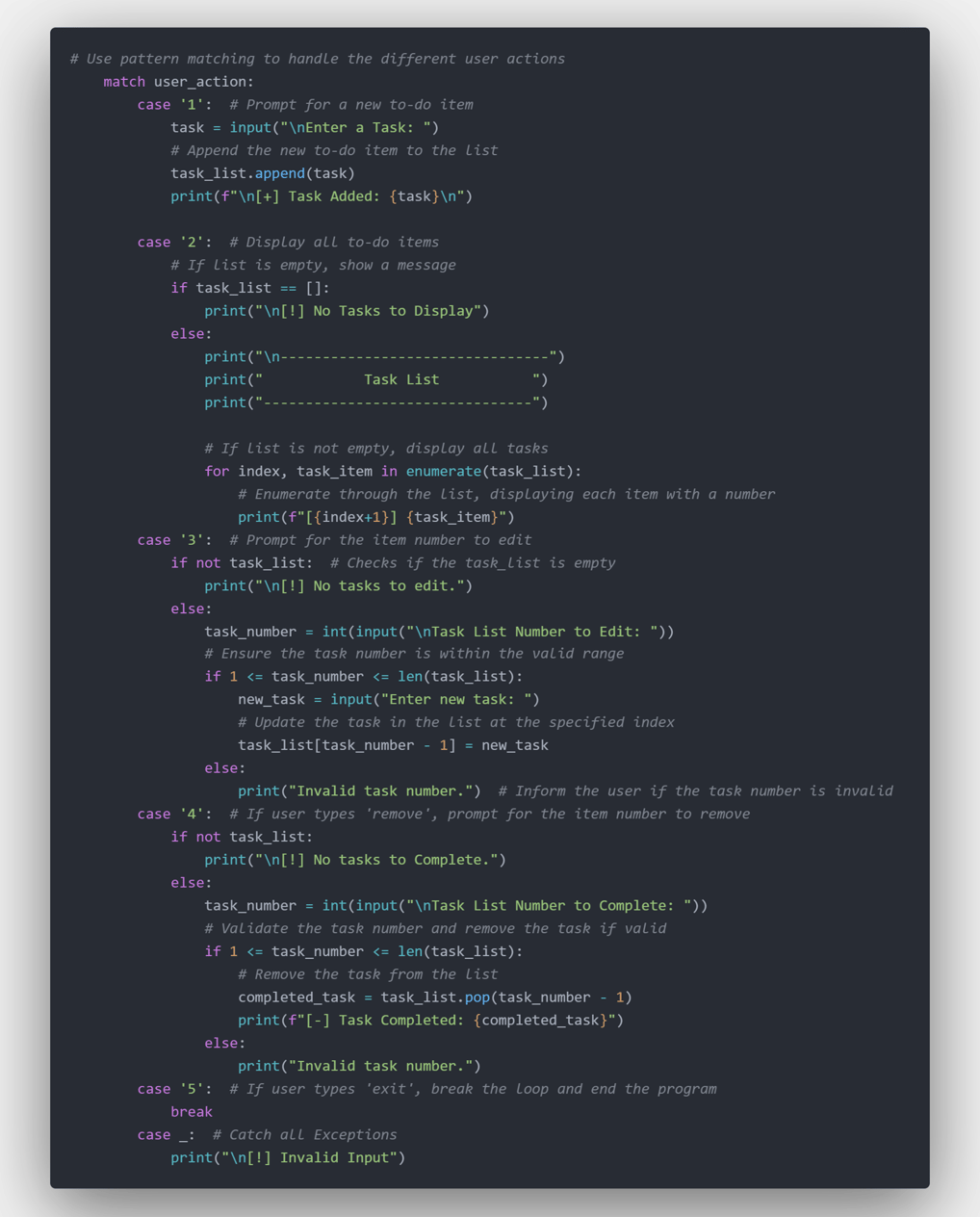
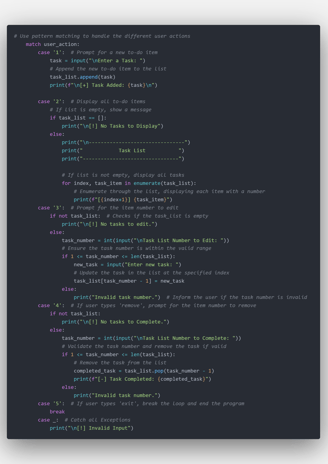
The ToDo App is built on the powerful 'match' statement feature introduced in Python 3.10 and later. This feature, akin to the switch case in other languages, is at the heart of the app's logic. It efficiently evaluates the user's input and matches it to the corresponding case, showcasing the app's modern design.
When the user enters '1', they are given the power to input a new task. This task is then added to the 'task_list,' and a confirmation message is displayed, giving the user a clear indication of their successful interaction with the app.
If the user enters '2', the program checks if 'task_list' is empty. If so, it displays "[!] No Tasks To Display". If not, it shows all tasks numerically.
If the user enters '3', the program first checks for tasks. If there are, it prompts for the number of the task to edit and then allows the user to input the new task name. If there are no tasks, it displays the message "[!] No tasks to edit." If the task number is not within the valid task number range, an error message reads "Invalid task number."
If the user enters '4', the program will remove a task from the 'task_list' after confirming the task number is valid and then display a completion message.
When the user enters '5', the program gracefully exits the infinite loop using the 'break' statement, effectively ending the program. This clear termination process ensures the app's lifecycle is well-defined and understood.
For handling unexpected inputs, the app uses a catch all case (_) that gracefully handles any unexpected inputs, informing the user that the input was invalid. This robust error-handling mechanism ensures a smooth user experience even in unforeseen circumstances, giving the user confidence in the app's reliability.
You can find the full code on my github page
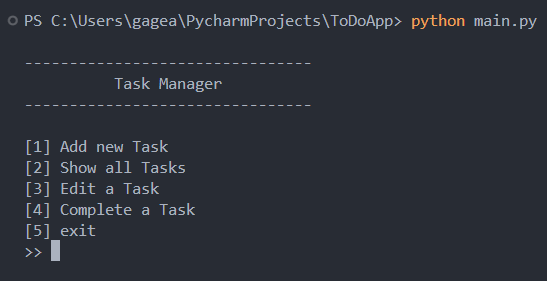
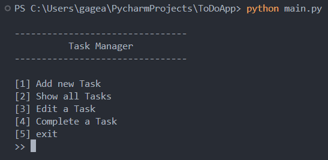
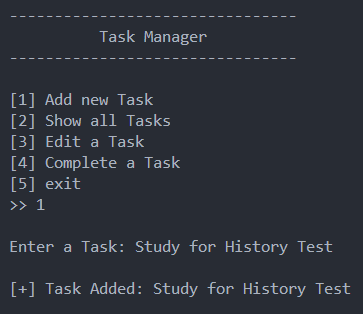
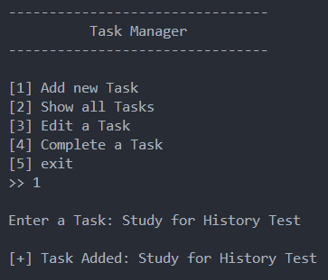
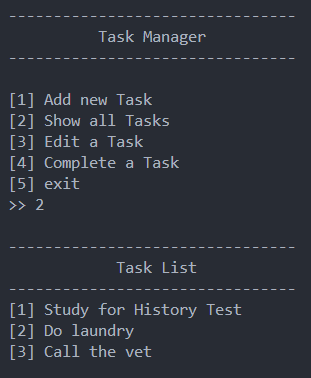
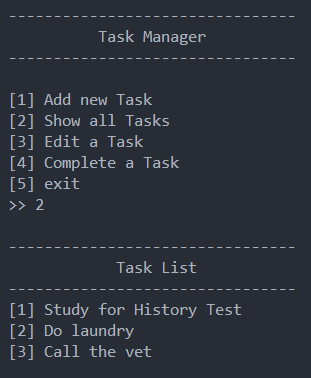
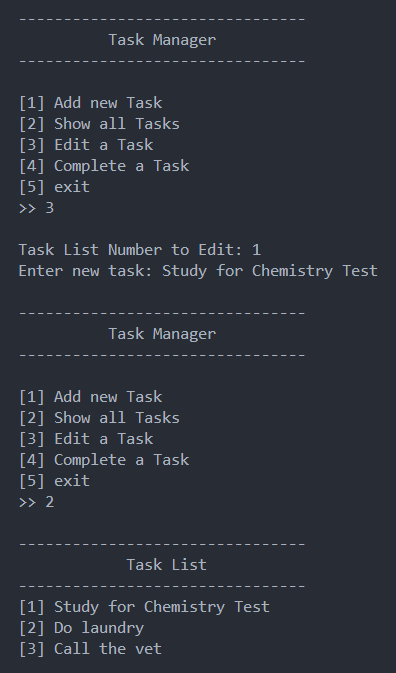
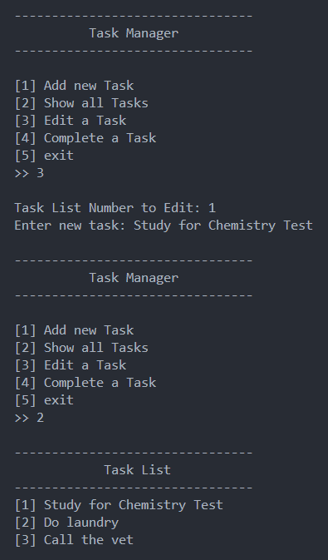